Drag Image From Web Page to Web Uploader
It's a known fact that file selection inputs are hard to style the mode developers want to, and then many but hide it and create a button that opens the file option dialog instead. Nowadays, though, we accept an even fancier way of treatment file selection: drag and drop.
Technically, this was already possible because most (if not all) implementations of the file choice input allowed you to drag files over it to select them, but this requires you to really testify the file
element. And so, let's really use the APIs given to us by the browser to implement a drag-and-driblet file selector and uploader.
In this commodity, we'll be using "vanilla" ES2015+ JavaScript (no frameworks or libraries) to complete this project, and it is assumed that y'all have a working knowledge of JavaScript in the browser. This example — bated from the ES2015+ syntax, which tin easily changed to ES5 syntax or transpiled by Boom-boom — should be compatible with every evergreen browser plus IE 10 and 11.
Here's a quick look at what you lot'll be making:
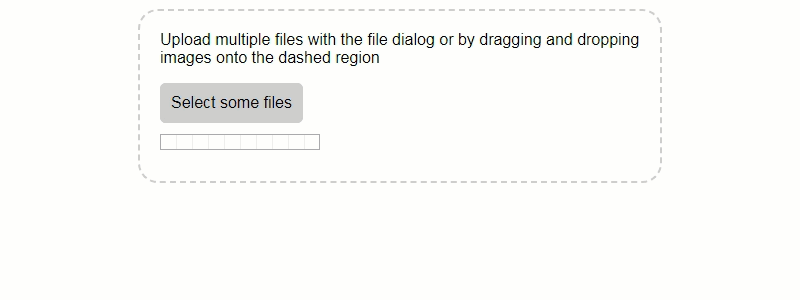
Drag-and-Drop Events
The commencement thing we demand to talk over is the events related to elevate-and-drop because they are the driving force backside this feature. In all, there are 8 events the browser fires related to drag and drop: drag
, dragend
, dragenter
, dragexit
, dragleave
, dragover
, dragstart
, and drop
. Nosotros won't be going over all of them because drag
, dragend
, dragexit
, and dragstart
are all fired on the element that is existence dragged, and in our case, we'll be dragging files in from our file organization rather than DOM elements, so these events will never popular up.
If you're curious about them, you can read some documentation near these events on MDN.
More than subsequently bound! Continue reading below ↓
As y'all might await, you lot can register consequence handlers for these events in the aforementioned mode yous register event handlers for most browser events: via addEventListener
.
permit dropArea = document.getElementById('drop-area') dropArea.addEventListener('dragenter', handlerFunction, faux) dropArea.addEventListener('dragleave', handlerFunction, false) dropArea.addEventListener('dragover', handlerFunction, false) dropArea.addEventListener('drib', handlerFunction, false)
Hither'due south a little table describing what these events do, using dropArea
from the code sample in gild to make the language clearer:
Consequence | When Is Information technology Fired? |
---|---|
dragenter | The dragged item is dragged over dropArea, making it the target for the drop event if the user drops information technology there. |
dragleave | The dragged particular is dragged off of dropArea and onto another element, making it the target for the drop upshot instead. |
dragover | Every few hundred milliseconds, while the dragged item is over dropArea and is moving. |
drib | The user releases their mouse button, dropping the dragged detail onto dropArea. |
Annotation that the dragged particular is dragged over a kid of dropArea
, dragleave
will fire on dropArea
and dragenter
will fire on that child chemical element because information technology is the new target
. The drop
event will propagate up to dropArea
(unless propagation is stopped past a different issue listener before information technology gets there), so it'll withal burn on dropArea
despite it not being the target
for the effect.
Also annotation that in society to create custom drag-and-drop interactions, you lot'll need to call outcome.preventDefault()
in each of the listeners for these events. If you don't, the browser will end up opening the file you lot dropped instead of sending it along to the driblet
event handler.
Setting Upward Our Form
Before nosotros start adding drag-and-drop functionality, nosotros'll need a basic form with a standard file
input. Technically this isn't necessary, only it'south a proficient idea to provide it equally an alternative in case the user has a browser without support for the drag-and-drop API.
<div id="drib-area"> <grade class="my-form"> <p>Upload multiple files with the file dialog or by dragging and dropping images onto the dashed region</p> <input type="file" id="fileElem" multiple accept="image/*" onchange="handleFiles(this.files)"> <label grade="button" for="fileElem">Select some files</label> </form> </div>
Pretty simple structure. You may find an onchange
handler on the input
. Nosotros'll have a await at that subsequently. Information technology would also be a good idea to add an activity
to the course
and a submit
button to assistance out those people who don't accept JavaScript enabled. And then you can use JavaScript to get rid of them for a cleaner form. In whatever case, you lot will need a server-side script to have the upload, whether it's something developed in-business firm, or yous're using a service like Cloudinary to practise it for y'all. Other than those notes, there's nothing special here, so permit's throw some styles in:
#driblet-area { border: 2px dashed #ccc; border-radius: 20px; width: 480px; font-family: sans-serif; margin: 100px auto; padding: 20px; } #drop-area.highlight { border-color: regal; } p { margin-top: 0; } .my-course { margin-lesser: 10px; } #gallery { margin-acme: 10px; } #gallery img { width: 150px; margin-bottom: 10px; margin-right: 10px; vertical-align: heart; } .button { display: inline-block; padding: 10px; groundwork: #ccc; cursor: pointer; edge-radius: 5px; edge: 1px solid #ccc; } .button:hover { background: #ddd; } #fileElem { display: none; }
Many of these styles aren't coming into play yet, but that'southward OK. The highlights, for at present, are that the file
input is hidden, but its characterization
is styled to look similar a push button, so people will realize they can click it to bring up the file pick dialog. Nosotros're also following a convention past outlining the drib area with dashed lines.
Calculation The Elevate-and-Drib Functionality
Now nosotros get to the meat of the situation: drag and drop. Let's throw a script in at the bottom of the page, or in a separate file, nonetheless you feel like doing it. The first thing nosotros need in the script is a reference to the drop surface area so nosotros tin can attach some events to it:
allow dropArea = document.getElementById('drop-area')
Now let's add together some events. We'll start off with calculation handlers to all the events to forestall default behaviors and finish the events from bubbles up any higher than necessary:
;['dragenter', 'dragover', 'dragleave', 'drib'].forEach(eventName => { dropArea.addEventListener(eventName, preventDefaults, false) }) role preventDefaults (e) { e.preventDefault() due east.stopPropagation() }
Now let's add an indicator to let the user know that they take indeed dragged the item over the correct area by using CSS to change the color of the border color of the drop area. The styles should already be in that location under the #drib-area.highlight
selector, so allow's use JS to add and remove that highlight
class when necessary.
;['dragenter', 'dragover'].forEach(eventName => { dropArea.addEventListener(eventName, highlight, simulated) }) ;['dragleave', 'drop'].forEach(eventName => { dropArea.addEventListener(eventName, unhighlight, false) }) function highlight(east) { dropArea.classList.add('highlight') } office unhighlight(due east) { dropArea.classList.remove('highlight') }
We had to use both dragenter
and dragover
for the highlighting because of what I mentioned earlier. If you commencement off hovering directly over dropArea
and then hover over 1 of its children, so dragleave
will exist fired and the highlight will exist removed. The dragover
event is fired subsequently the dragenter
and dragleave
events, then the highlight will be added back onto dropArea
before we see it beingness removed.
Nosotros besides remove the highlight when the dragged item leaves the designated expanse or when you lot drop the item.
Now all we need to practise is figure out what to do when some files are dropped:
dropArea.addEventListener('drop', handleDrop, false) function handleDrop(east) { let dt = due east.dataTransfer allow files = dt.files handleFiles(files) }
This doesn't bring united states anywhere near completion, but information technology does two important things:
- Demonstrates how to get the data for the files that were dropped.
- Gets u.s. to the same place that the
file
input
was at with itsonchange
handler: waiting forhandleFiles
.
Keep in listen that files
is not an assortment, merely a FileList
. So, when we implement handleFiles
, nosotros'll demand to convert information technology to an array in gild to iterate over it more than easily:
function handleFiles(files) { ([...files]).forEach(uploadFile) }
That was anticlimactic. Let'southward get into uploadFile
for the real meaty stuff.
office uploadFile(file) { permit url = 'YOUR URL Hither' permit formData = new FormData() formData.append('file', file) fetch(url, { method: 'POST', body: formData }) .so(() => { /* Done. Inform the user */ }) .take hold of(() => { /* Error. Inform the user */ }) }
Hither nosotros use FormData
, a congenital-in browser API for creating course information to send to the server. We so use the fetch
API to actually ship the epitome to the server. Make sure you lot modify the URL to work with your dorsum-finish or service, and formData.append
any boosted grade data yous may demand to give the server all the information information technology needs. Alternatively, if you want to support Internet Explorer, you may desire to employ XMLHttpRequest
, which means uploadFile
would wait like this instead:
role uploadFile(file) { var url = 'YOUR URL HERE' var xhr = new XMLHttpRequest() var formData = new FormData() xhr.open up('Postal service', url, true) xhr.addEventListener('readystatechange', function(e) { if (xhr.readyState == four && xhr.status == 200) { // Done. Inform the user } else if (xhr.readyState == 4 && xhr.status != 200) { // Error. Inform the user } }) formData.suspend('file', file) xhr.send(formData) }
Depending on how your server is fix up, yous may want to check for dissimilar ranges of status
numbers rather than simply 200
, but for our purposes, this volition work.
Additional Features
That is all of the base functionality, but ofttimes we want more functionality. Specifically, in this tutorial, we'll be calculation a preview pane that displays all the called images to the user, then nosotros'll add together a progress bar that lets the user see the progress of the uploads. So, allow's get started with previewing images.
Image Preview
There are a couple of ways you could do this: yous could await until after the paradigm has been uploaded and ask the server to send the URL of the epitome, but that means you need to wait and images can be pretty large sometimes. The alternative — which we'll be exploring today — is to use the FileReader API on the file data we received from the drib
event. This is asynchronous, and you could alternatively employ FileReaderSync, but we could exist trying to read several large files in a row, so this could block the thread for quite a while and actually ruin the experience. So let's create a previewFile
office and run into how it works:
function previewFile(file) { permit reader = new FileReader() reader.readAsDataURL(file) reader.onloadend = function() { allow img = certificate.createElement('img') img.src = reader.result document.getElementById('gallery').appendChild(img) } }
Here nosotros create a new FileReader
and phone call readAsDataURL
on it with the File
object. As mentioned, this is asynchronous, then we need to add an onloadend
result handler in order to get the result of the read. We then use the base 64 data URL as the src
for a new image element and add together it to the gallery
element. In that location are only two things that need to be washed to make this work now: add the gallery
chemical element, and brand certain previewFile
is really chosen.
Outset, add the following HTML right after the end of the grade
tag:
Nothing special; it's just a div. The styles are already specified for it and the images in it, and so there's zilch left to practise there. Now let's change the handleFiles
function to the post-obit:
function handleFiles(files) { files = [...files] files.forEach(uploadFile) files.forEach(previewFile) }
There are a few ways you could have done this, such as composition, or a single callback to forEach
that ran uploadFile
and previewFile
in it, but this works too. And with that, when yous drop or select some images, they should show upwardly nearly instantly below the form. The interesting thing virtually this is that — in certain applications — y'all may non really desire to upload images, but instead store the data URLs of them in localStorage
or some other client-side cache to be accessed by the app later. I tin't personally think of any good apply cases for this, but I'm willing to bet there are some.
Tracking Progress
If something might take a while, a progress bar can help a user realize progress is actually being made and give an indication of how long it will accept to be completed. Adding a progress indicator is pretty easy thanks to the HTML5 progress
tag. Let's start by adding that to the HTML lawmaking this fourth dimension.
<progress id="progress-bar" max=100 value=0></progress>
Y'all can plop that in right subsequently the label
or between the class
and gallery div
, whichever you fancy more. For that matter, y'all tin can place it wherever you desire within the torso
tags. No styles were added for this example, so information technology volition show the browser's default implementation, which is serviceable. Now allow's work on adding the JavaScript. We'll outset look at the implementation using fetch
and then nosotros'll show a version for XMLHttpRequest
. To start, we'll demand a couple of new variables at the acme of the script :
let filesDone = 0 permit filesToDo = 0 let progressBar = document.getElementById('progress-bar')
When using fetch
we're only able to decide when an upload is finished, and then the only information we track is how many files are selected to upload (as filesToDo
) and the number of files that have finished uploading (as filesDone
). We're also keeping a reference to the #progress-bar
chemical element then nosotros can update it speedily. Now let'due south create a couple of functions for managing the progress:
role initializeProgress(numfiles) { progressBar.value = 0 filesDone = 0 filesToDo = numfiles } function progressDone() { filesDone++ progressBar.value = filesDone / filesToDo * 100 }
When we offset uploading, initializeProgress
will be called to reset the progress bar. Then, with each completed upload, we'll call progressDone
to increase the number of completed uploads and update the progress bar to testify the current progress. So let's telephone call these functions past updating a couple of old functions:
function handleFiles(files) { files = [...files] initializeProgress(files.length) // <- Add this line files.forEach(uploadFile) files.forEach(previewFile) } part uploadFile(file) { let url = 'YOUR URL HERE' let formData = new FormData() formData.append('file', file) fetch(url, { method: 'Mail', torso: formData }) .then(progressDone) // <- Add `progressDone` call hither .catch(() => { /* Fault. Inform the user */ }) }
And that'due south it. Now let's take a look at the XMLHttpRequest
implementation. We could simply make a quick update to uploadFile
, but XMLHttpRequest
actually gives united states more functionality than fetch
, namely nosotros're able to add together an event listener for upload progress on each request, which will periodically give the states information well-nigh how much of the asking is finished. Because of this, nosotros need to track the percentage completion of each request instead of merely how many are done. So, allow's start with replacing the declarations for filesDone
and filesToDo
with the following:
let uploadProgress = []
Then we need to update our functions as well. We'll rename progressDone
to updateProgress
and change them to be the following:
function initializeProgress(numFiles) { progressBar.value = 0 uploadProgress = [] for(permit i = numFiles; i > 0; i--) { uploadProgress.push(0) } } function updateProgress(fileNumber, percentage) { uploadProgress[fileNumber] = percentage let full = uploadProgress.reduce((tot, curr) => tot + curr, 0) / uploadProgress.length progressBar.value = total }
Now initializeProgress
initializes an assortment with a length equal to numFiles
that is filled with zeroes, cogent that each file is 0% complete. In updateProgress
we find out which epitome is having their progress updated and modify the value at that alphabetize to the provided percent
. We then summate the full progress per centum by taking an average of all the percentages and update the progress bar to reverberate the calculated total. We still call initializeProgress
in handleFiles
the same as nosotros did in the fetch
example, so now all we need to update is uploadFile
to telephone call updateProgress
.
function uploadFile(file, i) { // <- Add together `i` parameter var url = 'YOUR URL Hither' var xhr = new XMLHttpRequest() var formData = new FormData() xhr.open up('Mail service', url, truthful) // Add post-obit event listener xhr.upload.addEventListener("progress", role(due east) { updateProgress(i, (e.loaded * 100.0 / e.full) || 100) }) xhr.addEventListener('readystatechange', office(e) { if (xhr.readyState == 4 && xhr.status == 200) { // Done. Inform the user } else if (xhr.readyState == 4 && xhr.condition != 200) { // Fault. Inform the user } }) formData.append('file', file) xhr.transport(formData) }
The showtime thing to note is that we added an i
parameter. This is the index of the file in the listing of files. Nosotros don't demand to update handleFiles
to laissez passer this parameter in because information technology is using forEach
, which already gives the alphabetize of the element as the second parameter to callbacks. Nosotros also added the progress
result listener to xhr.upload
and then we can call updateProgress
with the progress. The upshot object (referred to as e
in the code) has ii pertinent pieces of information on it: loaded
which contains the number of bytes that have been uploaded so far and total
which contains the number of bytes the file is in full.
The || 100
piece is in there considering sometimes if there is an error, due east.loaded
and e.total
will be nada, which ways the adding will come out as NaN
, so the 100
is used instead to report that the file is washed. Y'all could too use 0
. In either case, the error volition show up in the readystatechange
handler so that you tin can inform the user about them. This is simply to forbid exceptions from being thrown for trying to exercise math with NaN
.
Decision
That's the final piece. Yous now take a web folio where you can upload images via drag and drop, preview the images being uploaded immediately, and meet the progress of the upload in a progress bar. You can run into the final version (with XMLHttpRequest
) in action on CodePen, but be aware that the service I upload the files to has limits, so if a lot of people examination it out, it may break for a time.
(rb, ra, il)
wakelinwairespleet1990.blogspot.com
Source: https://www.smashingmagazine.com/2018/01/drag-drop-file-uploader-vanilla-js/